
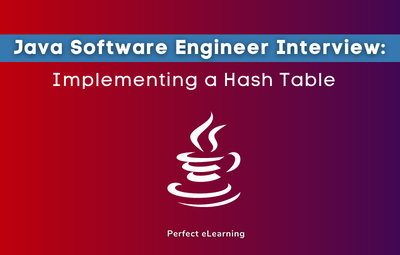
Are you a Java software engineer looking for a job? Do you want to ace your interview by showing off your skills in implementing a hash table? Look no further! In this article, we will guide you through the process of implementing a hash table in Java and give you some tips to help you prepare for your next interview.
A hash table is a data structure that stores key-value pairs. It is an efficient way to store and retrieve data because it allows constant time access to the data based on its key. In Java, a hash table is implemented using the Hashtable class or the more modern HashMap class.
How Hash Tables Work
A hash table works by taking a key and converting it into an index in an array. The value associated with that key is then stored at that index. The process of converting a key into an index is called hashing.
Designing a Hash Table
Before implementing a hash table, it is important to design it properly. A good hash table should have a large enough array to reduce the chances of collisions, but not too large that it wastes memory. The hashing function should also be designed to evenly distribute the keys across the array.
Implementing a Hash Table in Java
1. Creating the Hash Table Class:
To implement a hash table in Java, we first need to create a HashTable class. This class should have an array to store the key-value pairs and a hashing function to convert the keys into indices.
2. Adding Key-Value Pairs to the Hash Table:
To add a key-value pair to the hash table, we first hash the key to get its index in the array. We then store the value at that index. If there is already a value at that index, we have a collision.
3. Retrieving Values from the Hash Table:
To retrieve a value from the hash table, we hash the key to get its index in the array. We then retrieve the value at that index.
4. Removing Key-Value Pairs from the Hash Table:
To remove a key-value pair from the hash table, we first hash the key to get its index in the array. We then remove the value at that index.
Dealing with Collisions
Collisions occur when two or more keys hash to the same index in the array. To deal with collisions, we can use a technique called chaining. Chaining involves creating a linked list at each index in the array. When a collision occurs, we add the key-value pair to the linked list at that index.
Tips for Interview Success
When preparing for a Java software engineering interview, it is important to brush up on your data structure knowledge. Be sure to understand the basic concepts of hash tables, such as hashing and collisions. Practice implementing a hash table on your own and be ready to explain your thought process.
Conclusion
Implementing a hash table in Java is an essential skill for any software engineer. By understanding the basic concepts of hash tables and following the steps outlined in this article, you can confidently tackle any interview question related to hash tables.
Frequently Asked Questions (FAQs)
Q.What is the difference between Hashtable and HashMap in Java?
A.Hashtable is the older implementation of a hash table in Java and is synchronized, which makes it thread-safe. HashMap, on the other hand, is not synchronized, but is more efficient and can be used in multi-threaded environments with proper synchronization.
Q.What is the time complexity of accessing an element in a hash table?
A.In the best-case scenario, accessing an element in a hash table takes constant time, O(1). In the worst-case scenario, when there are many collisions, accessing an element can take linear time, O(n).
Q.Can a hash table have duplicate keys?
A.No, a hash table cannot have duplicate keys. If a key is added to the hash table and it already exists, the new value will replace the old value.
Q.What is the role of the hash code in a hash table?
A.The hash code is used to determine the index in the array where the key-value pair will be stored. A good hashing function should evenly distribute the keys across the array to minimize collisions.
Perfect eLearning is a tech-enabled education platform that provides IT courses with 100% Internship and Placement support. Perfect eLearning provides both Online classes and Offline classes only in Faridabad.
It provides a wide range of courses in areas such as Artificial Intelligence, Cloud Computing, Data Science, Digital Marketing, Full Stack Web Development, Block Chain, Data Analytics, and Mobile Application Development. Perfect eLearning, with its cutting-edge technology and expert instructors from Adobe, Microsoft, PWC, Google, Amazon, Flipkart, Nestle and Infoedge is the perfect place to start your IT education.
Perfect eLearning in Faridabad provides the training and support you need to succeed in today's fast-paced and constantly evolving tech industry, whether you're just starting out or looking to expand your skill set.
There's something here for everyone. Perfect eLearning provides the best online courses as well as complete internship and placement assistance.
Keep Learning, Keep Growing.
If you are confused and need Guidance over choosing the right programming language or right career in the tech industry, you can schedule a free counselling session with Perfect eLearning experts.